JavaScript
javascript 란?
javascript는 미국 선마이크로시스템스와 넷스케이프 커뮤니케이션스가 개발한 웹 브라우저에서 동작하는 스크립트 언어입니다.
- 클라이언트 기반의 객체지향 언어이고 가볍고 빠른 장점이 있습니다.
- 별도의 컴파일도 필요 없으며 변수들의 타입선언도 필요 없어 쉽게 배울 수 있습니다.
- 웹 문서를 동적으로 처리하며 이벤트( 키보드, 마우스, 클릭, 등 )를 사용하여 클라이언트 의 처리 기능을 주로 담당합니다.
프로그래밍 언어로, 스크립트 언어(Script Language)에 해당된다. 특수한 목적이 아닌 이상 모든 웹 브라우저에 인터프리터가 내장되어 있다. 오늘날 HTML, CSS와 함께 웹을 구성하는 요소의 하나다. HTML이 웹 페이지의 기본 구조를 담당하고, CSS가 디자인을 담당한다면 JavaScript는 클라이언트 단에서 웹 페이지가 동작하는 것을 담당한다.
Java와는 별 관계가 없는 언어이다. 얼핏 보기에는 문법이 Java와 비슷한데, 이는 Java와 Javascript 모두 C에서 영향받은 C-Family 언어이기 때문이다.
첫 탄생은 브랜던 아이크라는 사람이 10일만에 설계한 것으로부터 시작한다. 처음에는 Mocha라는 이름이었지만 4달 만에 LiveScript라는 이름으로 개명하고 다시 3달 후에는 JavaScript라는 이름이 되어 오늘날까지 이어지고 있다. Java와 구문이 유사하기도 하고 해서 이름을 JavaScript로 명명했다...는 표면상의 이유고 그 속은 Java의 유명세를 타서 묻어가려고 의도적으로 만든 것이다.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"/> </head> <body> <script> alert('Hello world'); </script> </body> </html> |
헬로월드 // 스크립트는 html에 <script></script> 태그 사이에 작성한다.
※ html에 파일 안에 작성함으로 파일명은 “ ~~.html ” 이다.
<!DOCTYPE html> <html> <body> <script src="myScript.js"></script> <!-- 외부에 작성된 javascript 파일을 불러오기. ~~.js 이다. --> </body> </html> |
javascript의 작성위치
보통은 그림 1처럼 head 영역에 작성하는데 여기에 작성하면 일단 html의 태그가 아닌 것을 따로 몰아서 작성할 수 있어 가독성이 좋아집니다. 사실 이 방법은 보통의 다른 언어들(C, JAVA...)의 작성방법과 유사합니다.(=함수를 상단에 작업함으로 써 이후 함수를 확인하기 쉽다.)
사실 javascript의 작성위치와 실행은 아무런 상관이 없습니다. 하지만 html5와 css3가 활성화 되고 있는 요즘에는 이전과 달리 javascript를 가지고 html 태그들을 컨트롤 할 경우가 많아졌습니다.
브라우져는 html코드를 위에서부터 순차적으로 렌더링 하여 화면에 출력합니다. 그러다 보니 javascript가 먼저 실행되어 버리면 가끔 아직 아래쪽에 렌더링 되지 않은 태그들을 javascript가 인식하지 못하여 실행되지 않거나 이상동작을 하는 경우가 발생합니다. 최근에는 그런 이유로 그림2의 방법을 추천하는 추세입니다.
JavaScript로 데이터를 화면에 출력하는 여러 방법
Writing into an HTML element, using innerHTML.
Writing into the HTML output using document.write().
Writing into an alert box, using window.alert().
Writing into the browser console, using console.log().
<!DOCTYPE html>
<html>
<body>
<h1>My First Web Page</h1>
<p>My First Paragraph</p>
<p id="demo"></p>
<button onclick="document.write(5 + 6)">Try it</button>
<script>
document.getElementById("demo").innerHTML = 5 + 6;
</script>
<script>
document.write(5 + 6);
</script>
<script>
window.alert(5 + 6);
</script>
<script>
console.log(5 + 6);
</script>
</body>
</html>
|
문제 : 계산기를 작성하여 각 버튼을 누르면 입력칸에 값이 출력되도록 해보자.
기본문법
코드의 끝은 꼭 세미콜론(;)으로 끝나야 합니다.
주석
// 한 줄 주석
/* 이건 더 긴,
여러 줄 주석입니다. */
Number – 실수와 정수
10.50
1001
String – 쌍 따옴표 또는 홑 따옴표
"John Doe"
'John Doe’
Boolean – 참 과 거짓
true, false
변수 선언 – var 또는 VAR
var x; //처음에 초기값을 정하지 않으면 undefined 값을 갖습니다.
x = 6;
null
null 값을 나타내는 특별한 키워드. JavaScript는 대소문자를 구분하므로, null은 Null, NULL 혹은 다른 변형과도 다릅니다.
배열
var coffees = ["French Roast", "Colombian", "Kona"];
// 차례대로 coffees[0],coffees[1], ...
상수 선언 const
const prefix = '212'; //읽기 전용 상수를 만들 수 있습니다. 상수 식별자의 구문은 변수 식별자와 같습니다. 문자, 밑줄이나 달러 기호로 시작해야 하고 문자, 숫자나 밑줄을 포함할 수 있습니다.
javascript 특수문자
- \0 Null Byte
- \b Backspace
- \f Form feed
- \n New line
- \r Carriage return
- \t Tab
- \v Vertical tab
- \' Apostrophe 혹은 작은 따옴표
- \" 큰 따옴표
- \\ 백슬래시
연산 - " + - * / % ++ -- "
(5 + 6) * 10
Operator
|
Example
|
Same As
|
=
|
x = y
|
x = y
|
+=
|
x += y
|
x = x + y
|
-=
|
x -= y
|
x = x - y
|
*=
|
x *= y
|
x = x * y
|
/=
|
x /= y
|
x = x / y
|
%=
|
x %= y
|
x = x % y
|
논리
Operator
|
Description
|
==
|
equal to
|
===
|
equal value and equal type
|
!=
|
not equal
|
!==
|
not equal value or not equal type
|
>
|
greater than
|
<
|
less than
|
>=
|
greater than or equal to
|
<=
|
less than or equal to
|
?
|
ternary operator
|
<!DOCTYPE html>
<html>
<body>
<script>
alert(1==2) //false
alert(1==1) //true
alert("one"=="two") //false
alert("one"=="one") //true
alert(1=='1'); //true
alert(1==='1'); //false
alert(null== undefined); //true
alert(null=== undefined); //false
alert(true== 1); //true
alert(true=== 1); //false
alert(true== '1'); //true
alert(true=== '1'); //false
alert(0 === -0); //true
alert(NaN === NaN); //false
</script>
</body>
</html>
|
※ null과 undefined는 값이 없다는 의미의 데이터 형이다. null은 값이 없음을 명시적으로 표시한 것이고, undefined는 그냥 값이 없는 상태라고 생각하자.
NaN은 0/0과 같은 연산의 결과로 만들어지는 특수한 데이터 형인데 숫자가 아니라는 뜻이다.
변수는 대소문자를 구별하고, 작성 끝엔 ; 을 붙입니다.
var lastname, lastName;
lastName = "Doe";
lastname = "Peterson";
|
메시지 창
* prompt : 변수 = prompt("메시지","초기값);
사용자로부터 입력을 받는 창으로 처음에는 '메시지'와 '초기값'이 출력된 상태로 나타나고, 사용자가 입력한 후 '확인'버튼이나 'Enter'키를 누르면 입력한 값이 변수에 기억된다. 그러나 사용자가 '취소'버튼을 누르거나 'ESC'키를 누르면 null값이 변수에 기억된다. 사용자가 입력한 값은 무조건 문자열로 저장된다.
* confirm : 변수 = confirm("메시지");
메시지를 출력한 창이 나타나고 사용자는 '확인(OK)'버튼 또는 '취소(cancel)'버튼을 선택할 수 있다. '확인'버튼을 누르면 true, '취소'버튼을 누르면 false가 변수에 기억된다.
* alert : alert("메시지");
메시지를 출력하는 창이 나타난다.
문서객체모델(DOM)
* 웹 브라우저가 HTML 페이지를 인식하는 방식(document 객체와 관련된 객체의 집합)
- 태그(Tag) : HTML 페이지에 존재하는 태그
- 문서 객체(DOM) : 태그를 자바스크립트에서 이용할 수 있는 객체
- 노드(Node) : HTML 페이지의 각 요소(html, head, body . . . 등)
- 요소 노드(Element Node) : HTML 태그
- 텍스트 노드(Text Node) : 요소 노드 안에 있는 글자
- 정적 문서 객체 생성 : HTML 페이지에 적혀 있는 태그들을 읽으며 생성하는 것
- 동적 문서 객체 생성 : JavaScript로 원래 HTML 페이지에는 없던 문서 객체를 생성하는 것
* html 태그를 그대로 화면에 출력하고자 할 때는 textContent를 사용한다.
함수 (Function)
function 함수명(매개변수, 매개변수,...) { 실행 구문}
|
※ 따로 써야하는 반환형, 비반환형 은 없으며, 만약 값을 돌려 받길 원한다면 마지막에 “return 값” 형태를 써주기만 하면 된다.
function tooHigh() { alert(“하늘을 난다“);} |
function mirror() { var x = 5+3; return x;} |
<body>
<p id="demo"></p>
<script>
function myFunction(a, b) {
return a * b;
}
document.getElementById("demo").innerHTML = myFunction(4, 3);
</script>
</body>
|
<body>
<p id="demo"></p>
<script>
function myFunction() {
document.getElementById("demo").innerHTML = "Hello World!";
}
myFunction();
</script>
</body>
|
문제 : 10과 5의 더한 값, 뺀 값, 곱한 값, 나눈 값을 출력 하는 버튼을 각각 만들자.(4개)
이벤트
Event
|
Description
|
onchange
|
An HTML element has been changed
|
onclick
|
The user clicks an HTML element
|
onmouseover
|
The user moves the mouse over an HTML element
|
onmouseout
|
The user moves the mouse away from an HTML element
|
onkeydown
|
The user pushes a keyboard key
|
onload
|
The browser has finished loading the page
|
<!DOCTYPE html>
<html>
<body>
<p>Select a new car from the list.</p>
<select id="mySelect" onchange="myFunction()">
<option value="Audi">Audi
<option value="BMW">BMW
<option value="Mercedes">Mercedes
<option value="Volvo">Volvo
</select>
<p>When you select a new car, a function is triggered which outputs the value of the selected car.</p>
![]() <p id="demo"></p>
<script>
function myFunction() {
var x = document.getElementById("mySelect").value;
document.getElementById("demo").innerHTML = "You selected: " + x;
}
</script>
</body>
</html>
|
문제 : 다음 페이지의 onmouseover 속성의 값을 참조하여 위에 그림처럼 만들고 선택을 하면 “글자색” 의 색이 선택한 색상으로 바뀌게 하자.
<!DOCTYPE html>
<html>
<body>
<span onmouseover="this.style.color='red'">Mouse over me!</span>
</body>
</html>
|
<!DOCTYPE html>
<html>
<head>
<style>
div {
width: 200px;
height: 100px;
border: 1px solid black;
}
</style>
</head>
<body>
<div onmousemove="myFunction(event)" onmouseout="clearCoor()"></div>
<p>마우스를 위에 네모상자에 올리면 네모 상자 안에서의 위치를 알려줍니다.</p>
<p id="demo"></p>
<script>
function myFunction(e) {
var x = e.clientX;
var y = e.clientY;
var coor = "Coordinates: (" + x + "," + y + ")";
document.getElementById("demo").innerHTML = coor;
}
function clearCoor() {
document.getElementById("demo").innerHTML = "";
}
</script>
</body>
</html>
|
<!DOCTYPE html>
<html>
<body>
<p>A function is triggered when the user is pressing a key in the input field.</p>
<input type="text" onkeydown="myFunction(event)">
<script>
function myFunction(e) {
alert(e.key);
}
</script>
</body>
</html>
|
<!DOCTYPE html>
<html>
<body onload="myFunction()">
<h1>Hello World!</h1>
<script>
function myFunction() {
alert("Page is loaded");
}
</script>
</body>
</html>
|
<!DOCTYPE html>
<html>
<body onload="myFunction()">
<script>
function myFunction() {
var tempUser = navigator.userAgent;
// userAgent 값에 iPhone, iPad, ipot, Android 라는 문자열이 하나라도 존재한다면 모바일로 간주됨.
if (tempUser.indexOf("iPhone") > 0 || tempUser.indexOf("iPad") > 0
|| tempUser.indexOf("iPot") > 0 || tempUser.indexOf("Android") > 0) {
alert("모바일 페이지");
} else {
alert("데스크탑 페이지");
}
}
</script>
</body>
</html>
|
/* 6페이지 문제 정답 */
<!DOCTYPE html>
<html>
<body>
<p>Select a new car from the list.</p>
<select id="mySelect" onchange="myFunction()">
<option>= 색상을 선택하세요 =</option>
<option value="red">빨간색</option>
<option value="blue">파란색</option>
<option value="green">녹색</option>
</select>
<p id="demo">글자색</p>
<script>
function myFunction() {
var x = document.getElementById("mySelect").value;
document.getElementById("demo").style.color=x;
}
</script>
</body>
</html>
|
내장함수
String 관련함수
설명
|
예제
|
문자열의 길이를 가져온다.
|
var txt = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
var sln = txt.length;
|
문자의 위치를 가져온다.
|
var str = "Please locate where 'locate' occurs!";
var pos = str.indexOf("locate");
|
문자의 위치를 뒤에서 가져온다.
|
var str = "Please locate where 'locate' occurs!";
var pos = str.lastIndexOf("locate");
|
문자의 위치를 특정위치에서부터 찾는다.
|
var str = "Please locate where 'locate' occurs!";
var pos = str.indexOf("locate",15);
|
문자를 자른다. (시작위치, 끝위치)
|
var str = "Apple, Banana, Kiwi";
var res = str.slice(7, 13);
|
문자를 자른다. (시작위치, 끝위치)
|
var str = "Apple, Banana, Kiwi";
var res = str.substring(7, 13);
|
문자를 특정위치에서부터 개수만큼 가져온다.
|
var str = "Apple, Banana, Kiwi";
var res = str.substr(7, 6);
|
글자 바꾸기(첫 번째 것만 바꿈)
|
str = "Please visit Microsoft!";
var n = str.replace("Microsoft", "W3Schools");
|
글자 바꾸기(전체 다 바꿈)
|
str = "Please visit Microsoft and Microsoft!";
var n = str.replace(/Microsoft/g, "W3Schools");
|
대소문자를 구별한다.
|
str = "Please visit Microsoft!";
var n = str.replace("MICROSOFT", "W3Schools");
|
대소문자 구별 안함.
|
str = "Please visit Microsoft!";
var n = str.replace(/MICROSOFT/i, "W3Schools");
|
소문자로 변경
|
var text1 = "Hello World!"; // String
var text2 = text1.toLowerCase(); // converted to lower
|
대문자로 변경
|
var text1 = "Hello World!"; // String
var text2 = text1.toUpperCase(); // converted to upper
|
글자 합치기
|
var text1 = "Hello";
var text2 = "World";
var text3 = text1.concat(" ", text2);
|
글자 합치기
|
var text = "Hello" + " " + "World!";
var text = "Hello".concat(" ", "World!"); // same as
|
특정위치 한 개의 글자 가져오기
|
var str = "HELLO WORLD";
str.charAt(0); // returns H
|
특정위치 글자의 KeyCode 가져오기
|
var str = "HELLO WORLD";
str.charCodeAt(0); // returns 72
|
특정위치 한 개의 글자 가져오기
|
var str = "HELLO WORLD";
str[0]; // returns H
|
글자 특정 문자 기준으로 나누기
|
var txt = "a,b,c,d,e"; // String
txt.split(","); // Split on commas
txt.split(" "); // Split on spaces
txt.split("|"); // Split on pipe
|
글자를 하나씩 나누기
|
var txt = "Hello"; // String
txt.split(""); // Split in characters
|
문제 : ses의 노래 “샤랄라”에서 “샤랄라라”는 몇 번 나올까?
문제 : 터틀맨의 “빙고”에서 가사 각 구절 첫 번째 글자만 가져와서 출력하시오.
Number 관련함수
숫자를 문자로
|
var x = 123;
x.toString(); // returns 123 from variable x
(123).toString(); // returns 123 from literal 123
|
지수
매개변수는 표기 자릿수
|
var x = 9.656;
x.toExponential(2); // returns 9.66e+0
x.toExponential(4); // returns 9.6560e+0
x.toExponential(6); // returns 9.656000e+0
|
소수의 자릿수 제한
|
var x = 9.656;
x.toFixed(0); // returns 10
x.toFixed(2); // returns 9.66
x.toFixed(4); // returns 9.6560
|
수의 길이를 제한
|
var x = 9.656;
x.toPrecision(); // returns 9.656
x.toPrecision(2); // returns 9.7
x.toPrecision(4); // returns 9.656
|
수의 원시 데이터형(primitive value)을 리턴
|
x = new Number(10);
alert(x.valueOf()); // number, 10
alert(typeof x); // object
alert(typeof x.valueOf()); // number
|
객체의 값을 숫자로 바꿈
|
x = true;
Number(x); // returns 1
x = false;
Number(x); // returns 0
x = new Date();
Number(x); // returns 1404568027739
Used on Date(), the Number() method returns the number of milliseconds since 1.1.1970.
x = "10"
Number(x); // returns 10
|
첫 번째 문자를 숫자로 바꿈
|
parseInt("10"); // returns 10
parseInt("10.33"); // returns 10
parseInt("10 20 30"); // returns 10
parseInt("10 years"); // returns 10
|
첫 번째 문자를 실수 일때만 실수로 바꿈
|
parseFloat("10"); // returns 10
parseFloat("10.33"); // returns 10.33
parseFloat("10 20 30"); // returns 10
|
Property
|
Description
|
MAX_VALUE
|
Returns the largest number possible in JavaScript
|
MIN_VALUE
|
Returns the smallest number possible in JavaScript
|
NaN
|
Represents a "Not-a-Number" value
|
Math 클래스
파이
|
Math.PI;
|
반올림
|
Math.round(4.7); // returns 5
Math.round(4.4); // returns 4
|
승수 (숫자, 승수)
|
Math.pow(8, 2); // returns 64
|
루트
|
Math.sqrt(64); // returns 8
|
절대 값
|
Math.abs(-4.7); // returns 4.7
|
올림
|
Math.ceil(4.4); // returns 5
|
내림
|
Math.floor(4.7); // returns 4
|
사인
|
Math.sin(90 * Math.PI / 180);
// returns 1 (the sine of 90 degrees)
|
코사인
|
Math.cos(0 * Math.PI / 180);
// returns 1 (the cos of 0 degrees)
|
최소 값
|
Math.min(0, 150, 30, 20, -8, -200); // returns -200
|
최대 값
|
Math.max(0, 150, 30, 20, -8, -200); // returns 150
|
0이상 1미만의 랜덤 값 출력
|
Math.random(); // returns a random number
예제)
Math.floor(Math.random() * 10); // 0~9 사이의 랜덤
Math.floor(Math.random() * 11); // 0~10 사이의 랜덤
Math.floor(Math.random() * 10) + 1; //1~10 사이의 랜덤
|
Date 클래스
new Date()
new Date(milliseconds)
new Date(dateString)
new Date(year, month, day, hours, minutes, seconds, milliseconds)
<script>
var d = new Date(86400000);
document.getElementById("demo").innerHTML = d;
//Fri Jan 02 1970 09:00:00 GMT+0900 (대한민국 표준시)
</script>
|
<script>
var d = new Date(99, 5, 24, 11, 33, 30, 0);
document.getElementById("demo").innerHTML = d;
//Thu Jun 24 1999 11:33:30 GMT+0900 (대한민국 표준시)
</script>
|
Date 클래스 입력
Type
|
Example
|
ISO Date
|
"2015-03-25" (The International Standard)
|
Short Date
|
"03/25/2015"
|
Long Date
|
"Mar 25 2015" or "25 Mar 2015"
|
Full Date
|
"Wednesday March 25 2015"
|
Date 관련함수
Method
|
Description
|
getDate()
|
Get the day as a number (1-31)
|
getDay()
|
Get the weekday as a number (0-6)
|
getFullYear()
|
Get the four digit year (yyyy)
|
getHours()
|
Get the hour (0-23)
|
getMilliseconds()
|
Get the milliseconds (0-999)
|
getMinutes()
|
Get the minutes (0-59)
|
getMonth()
|
Get the month (0-11)
|
getSeconds()
|
Get the seconds (0-59)
|
getTime()
|
Get the time (milliseconds since January 1, 1970)
|
<!DOCTYPE html>
<html>
<body>
<p>The getDay() method returns the weekday as a number:</p>
<p id="demo"></p>
<script>
var d = new Date();
document.getElementById("demo").innerHTML = d.getDay();
</script>
</body>
</html>
|
배열
var cars = ["Saab", "Volvo", "BMW"];
var cars = new Array("Saab", "Volvo", "BMW"); // 가능 하지만 쓰지 않기를 바람.
배열 관련함수
배열을 문자로
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits.toString();
// return Banana,Orange,Apple,Mango
|
배열을 join의 매개변수로 결합
|
var fruits = ["Banana", "Orange","Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits.join(" * ");
// return Banana * Orange * Apple * Mango
|
마지막 배열 삭제
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.pop(); // Removes the last element ("Mango") from fruits
|
삭제한 배열을 변수에 담을 수 있다.
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
var x = fruits.pop(); // the value of x is "Mango“
|
마지막에 새 배열 추가
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.push("Kiwi"); // Adds a new element ("Kiwi") to fruits
|
첫 번째 배열 삭제
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.shift(); // Removes the first element "Banana" from fruits
|
첫 번째 배열 추가
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.unshift("Lemon"); // Adds a new element "Lemon" to fruits
|
마지막에 새 배열 추가
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits[fruits.length] = "Kiwi"; // Appends "Kiwi" to fruit
|
특정 위치의 배열 삭제
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
delete fruits[0]; // Changes the first element in fruits to undefined
|
splice(위치, 삭제 개수, 추가할 값)
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.splice(2, 0, "Lemon", "Kiwi");
|
splice(위치, 삭제할 개수)
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.splice(0, 1); // Removes the first element of fruits
|
배열 2개 합치기
|
var myGirls = ["Cecilie", "Lone"];
var myBoys = ["Emil", "Tobias","Linus"];
var myChildren = myGirls.concat(myBoys);
// Concatenates (joins) myGirls and myBoys
|
배열 3개 합치기
|
var arr1 = ["Cecilie", "Lone"];
var arr2 = ["Emil", "Tobias","Linus"];
var arr3 = ["Robin", "Morgan"];
var myChildren = arr1.concat(arr2, arr3);
// Concatenates arr1 with arr2 and arr3
|
배열 잘라내기 (위치부터)
|
var fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
var citrus = fruits.slice(3);
|
배열 잘라내기(시작위치, 끝위치)
|
var fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
var citrus = fruits.slice(1, 3);
|
배열정렬. 오름차순, 내림차순
|
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.sort(); // Sorts the elements of fruits
fruits.reverse(); // Reverses the order of the elements
|
switch 문
switch(expression) {
case n:
code block
break;
case n:
code block
break;
default:
code block
}
|
for 문
for (statement 1; statement 2; statement 3) {
code block to be executed
}
|
while 문
while (condition) {
code block to be executed
}
|
do/while 문
do {
code block to be executed
}
while (condition);
|
예약어
stract
|
arguments
|
await*
|
boolean
|
break
|
byte
|
case
|
catch
|
char
|
class*
|
const
|
continue
|
debugger
|
default
|
delete
|
do
|
double
|
else
|
enum*
|
eval
|
export*
|
extends*
|
false
|
final
|
finally
|
float
|
for
|
function
|
goto
|
if
|
implements
|
import*
|
in
|
instanceof
|
int
|
interface
|
let*
|
long
|
native
|
new
|
null
|
package
|
private
|
protected
|
public
|
return
|
short
|
static
|
super*
|
switch
|
synchronized
|
this
|
throw
|
throws
|
transient
|
true
|
try
|
typeof
|
var
|
void
|
volatile
|
while
|
with
|
yield
|
Form 태그
<!DOCTYPE html>
<html>
<head>
<script>
function getValue(){
alert(document.myForm.fname.value);
// document.myForm.fname.value="하이";
}
</script>
</head>
<body>
<form name="myForm" onsubmit="getValue()">
Name: <input type="text" name="fname">
<input type="submit" value="전송">
</form>
</body>
</html>
|
※ form의 경우 name 으로 객체 접근이 가능 하다. name으로 접근할 경우 DOM Tree로 자식 객체에 접근해야 한다.
※ onsubmit() 는 submit 버튼을 누를 때 발생하며 이 이벤트는 submit 버튼이 아닌 form 에 주어야 한다.
<!DOCTYPE html>
<html>
<head>
<script>
function getValue(){
document.myForm.action="js08.html";
document.myForm.submit();
}
</script>
</head>
<body>
<form name="myForm">
Name: <input type="text" name="fname">
<input type="button" value="전송" onclick="getValue()">
</form>
</body>
</html>
|
※ form 속성의 action 이나 submit 버튼의 기능들을 JavaScript 코드로 재 설정 가능하다.
Link
※ JavaScript를 이용하여 링크를 바꿀수도 있다. 링크는 submit과 달라서 페이지 이동 시 기본적으록 가져가는 값이 없다.
<!DOCTYPE html>
<html>
<head>
<script>
function goDaum(){
location.href="http://daum.net";
}
</script>
</head>
<body>
<form name="myForm">
<input type="text" name="fname">
<input type="button" value="다음" onclick="goDaum()">
<input type="button" value="네이버" onclick="location.href='http://naver.com'">
</form>
</body>
</html>
|
※ Link에서 값을 넘기는 방법
http://웹주소?name1=값&name2=값&name3=값&....
ex)
https://search.naver.com/search.naver?query=coffee
· ? - 주소 이후 넘길 name을 쓰기 위해서 무조건 써야한다.
· name1 = 값 – name1은에서 name으로 설정한 그 이름이다.
· & - name이 여러개 일 경우에 연결자 이다.
추가입력
var person = prompt("Please enter your name", "Harry Potter");
|
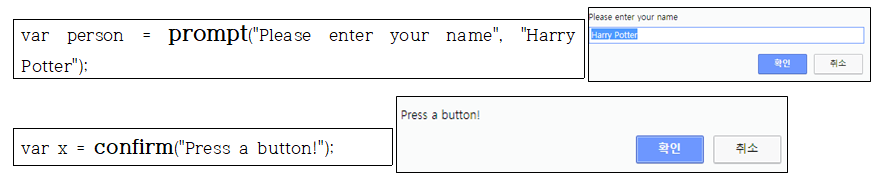
새창(팝업창) 띄우기
<!DOCTYPE html>
<html>
<head>
<script>
function goDaum(){
window.open("http://daum.net",'_blank','toolbar=no,location=no,status=no,menubar=no,scrollbars=auto,resizable=no,directories=no,width=400,height=400,top=100,left=100');
}
</script>
</head>
<body>
<form name="myForm">
<input type="text" name="fname">
<input type="button" value="다음" onclick="goDaum()">
</form>
</body>
</html>
|
새창 띄우기 속성
페이지 이동1 // index.html
<!DOCTYPE html>
<html>
<head>
<script>
function goNext(){
location.href="subPage.html";
}
</script>
</head>
<body>
<input type="button" value="다음페이지" onclick="goNext()">
</form>
</body>
</html>
|
페이지 이동2 // subPage.html
<!DOCTYPE html>
<html>
<head>
<script>
function goPrev(){
location.href="index.html";
// history.go(-1);
// history.back();
}
</script>
</head>
<body>
<input type="button" value="돌아가기" onclick="goPrev()">
</form>
</body>
</html>
|
back()은 바로 전 단계로만 가고, go()의 경우 매게변수를 통해 이전과 이후로 페이지를 바꿀 수 있다.
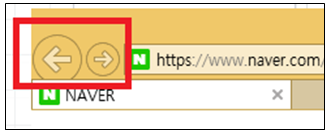
브라우져 창에서 위의 그림의 버튼과 같은 기능이다.
보통의 경우 취소를 누를 경우 <input type=“reset”>
대신에 뒤로 가기 처리를 많이한다.
예제
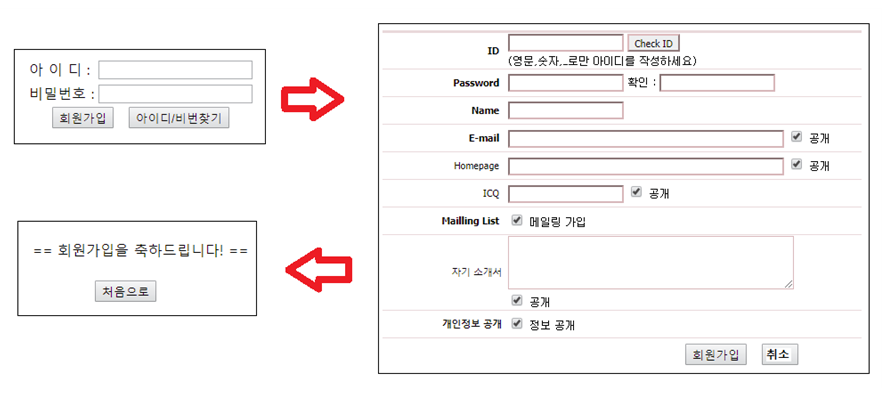
2. 적절히 link와 submit를 활용해서 작성.
3. main에서 회원가입을 눌렀을 경우 회원가입 페이지로 이동.
4. 회원가입 페이지에서 회원가입 버튼을 눌렀을 경우
- 빈칸체크
- 비밀번호 와 확인의 일치 여부 체크
- 아이디는 8자 이하
- 비밀번호는 12자 이하, 반드시 숫자와 영문 혼합
- e메일 형식 체크
- 이상 없을 시 입력한 전체 내용을 alert()으로 보여줌.
- 확인을 누를 시 성공 페이지로
- 하단의 취소를 누르면 메인 페이지로 이동
------
'IT와 코딩' 카테고리의 다른 글
나도 드디어 맥북에어 유저가 되었다 (1) | 2022.09.20 |
---|---|
맥북에어 M1 장고(django) 설치 순서 (3) | 2022.09.20 |
AWS 유분투에 그누보드 올려보기 (0) | 2022.09.18 |
피온4 감독모드 파이썬으로 초보자도 매크로를 만들어 보자 pyautogui 와 pydirectinput (4) | 2022.09.11 |
python 3.9 버전 cuda 설치하여 pytorch 연동하기 (0) | 2022.09.09 |
댓글